
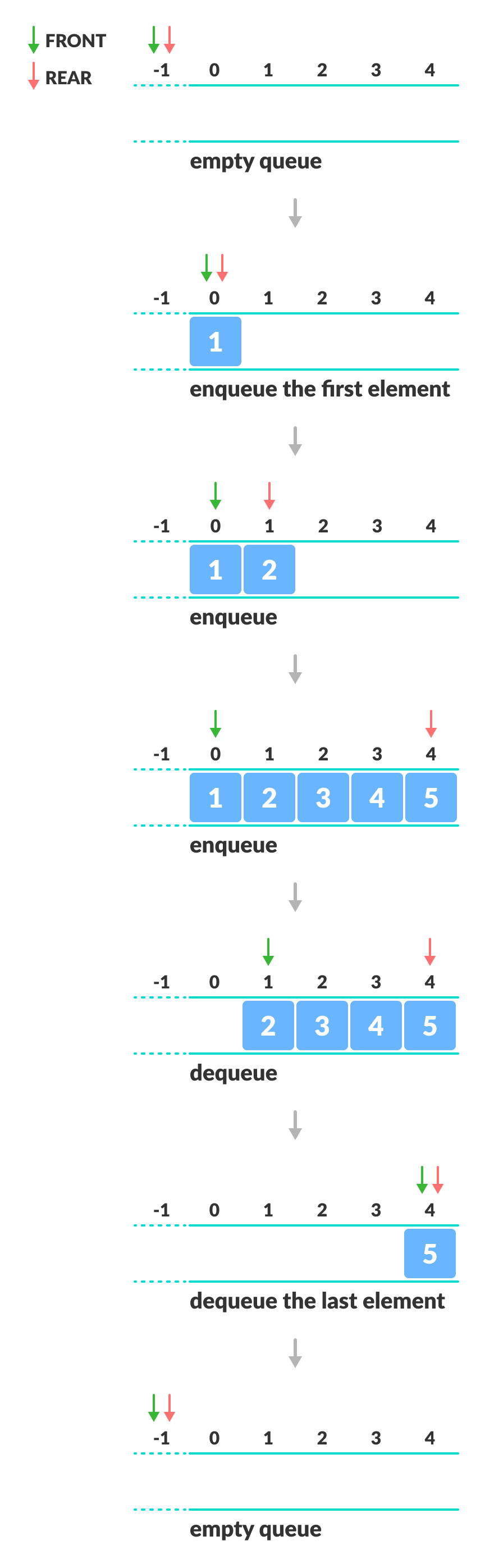
Now add the following code that calls heappop (…) 100 times to remove all assignments from the queue and print out their names including their priority value: for count in range(1,101):Īs you can see, by using heappop (…) we indeed get the assignments in the right order from the queue. The list is actually a “flattened” representation of a binary tree, the data structure that heapq is using to make the push and pop operations as efficient as possible, while making sure that heappop (…) always gives you the lowest value element from the queue. However, the list also does not reflect the order in which the assignments have been added to the queue.
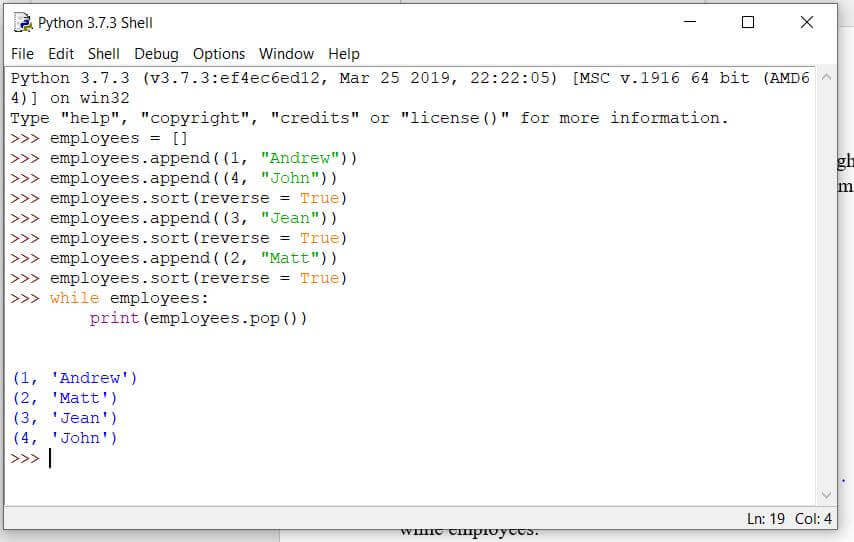
When you look at the output produced by the print statement in the last line, you may be disappointed because it doesn’t look like the list is really ordered based on the priority numbers of the assignments. Heapq.heappush(pQueue, 'Assignment ' + str(priority)) The code starts with an empty list in variable pQueue and then simulates the arrival of 100 assignments with random priority using heappush (…) to add a new assignment to the queue. The reason we defined the highest priority to be given by the number 1 and the lowest priority by the number 9 is that heapq implements a min heap in which heappop (…) always returns the lowest value element according to the < relation in contrast to a max heap in which heappop (…) would always return the highest value element. “Assignment 1” < “Assignment 2” < … < “Assignment 9”. In the following code, we again use strings for representing our assignments and encode the priority in the strings themselves so that their lexicographical order corresponds to their priority, i.e. The heapq module among other things provides a set of functions for adding elements to a list (function heappush (…)) and for removing the first item with highest priority (function heappop (…)). That means we need to make sure we keep the assignments in the queue ordered based on their priority so that taking the first assignment from the queue will be that with the highest priority. Let’s say that instead of performing the assignments in the order in which they arrive (first-in-first-out), the assignments have a priority value between 1 and 9 with 1 meaning highest and 9 meaning lowest priority. The idea of a priority queue is that items in the collection are always kept ordered based on their < relation so that when we take the first item from the queue it will always be the one with lowest (or highest) value.įor instance, let’s get back to the example we started this section with of managing a queue of assignments or tasks that need to be performed. task_done () print ( f ' seconds' ) asyncio. sleep ( sleep_for ) # Notify the queue that the "work item" has been processed. get () # Sleep for the "sleep_for" seconds. Import asyncio import random import time async def worker ( name, queue ): while True : # Get a "work item" out of the queue. Queues can be used to distribute workload between several QueueFull ¶Įxception raised when the put_nowait() method is called This exception is raised when the get_nowait() method LifoQueue ¶Ī variant of Queue that retrieves most recently addedĮntries first (last in, first out). PriorityQueue ¶Ī variant of Queue retrieves entries in priority order Raises ValueError if called more times than there were Items have been processed (meaning that a task_done()Ĭall was received for every item that had been put() If a join() is currently blocking, it will resume when all Queue that the processing on the task is complete. For each get() used toįetch a task, a subsequent call to task_done() tells the Indicate that a formerly enqueued task is complete.
Get priority queue python free#
If no free slot is immediately available, raise QueueFull. Put an item into the queue without blocking. If the queue is full, wait until aįree slot is available before adding the item. Task_done() to indicate that the item was retrieved and all The count goes down whenever a consumer coroutine calls The count of unfinished tasks goes up whenever an item is added Return an item if one is immediately available, else raiseīlock until all items in the queue have been received and processed. Remove and return an item from the queue. If the queue was initialized with maxsize=0 (the default), Return True if there are maxsize items in the queue. Return True if the queue is empty, False otherwise. Changed in version 3.10: Removed the loop parameter.
